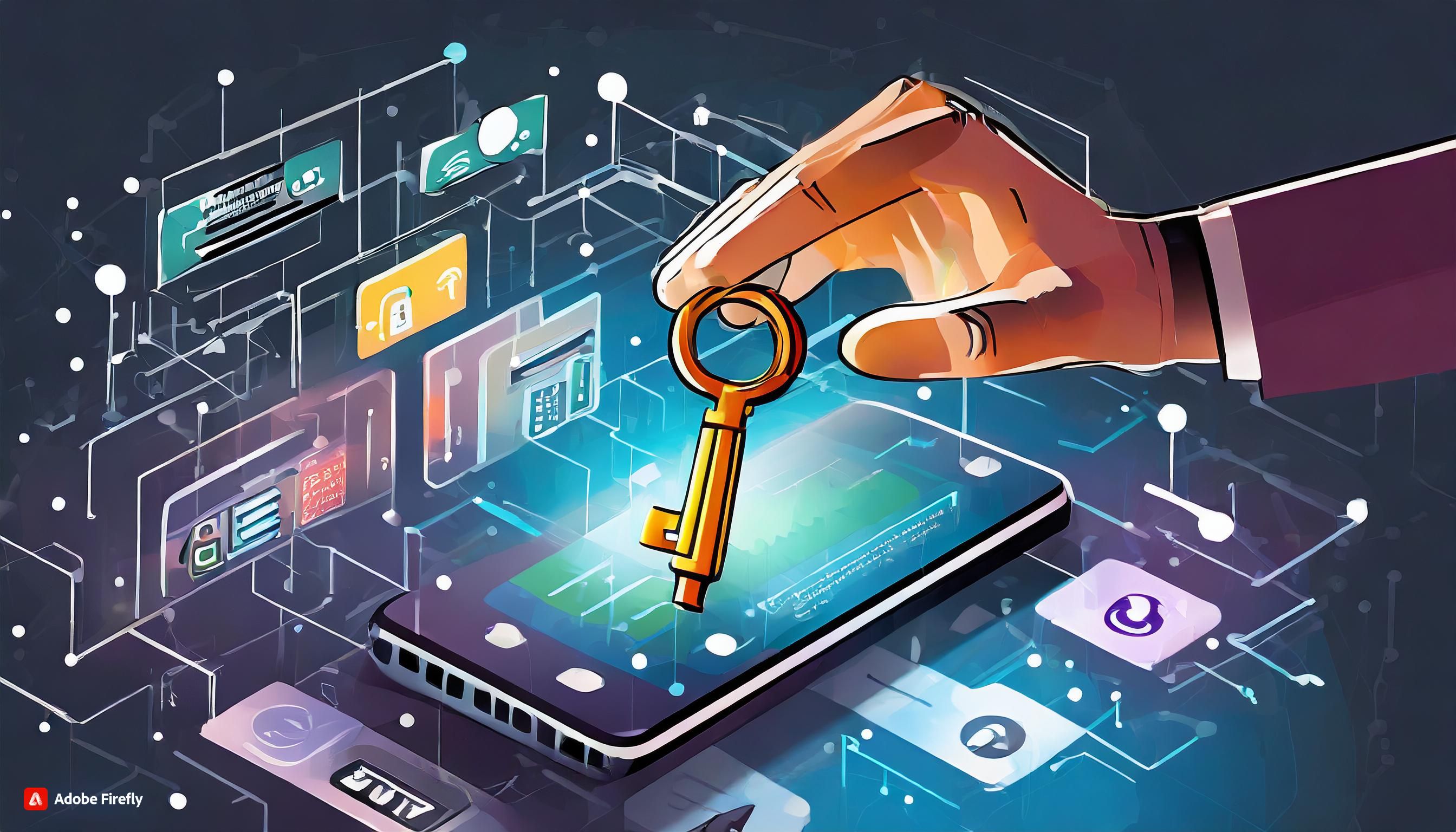
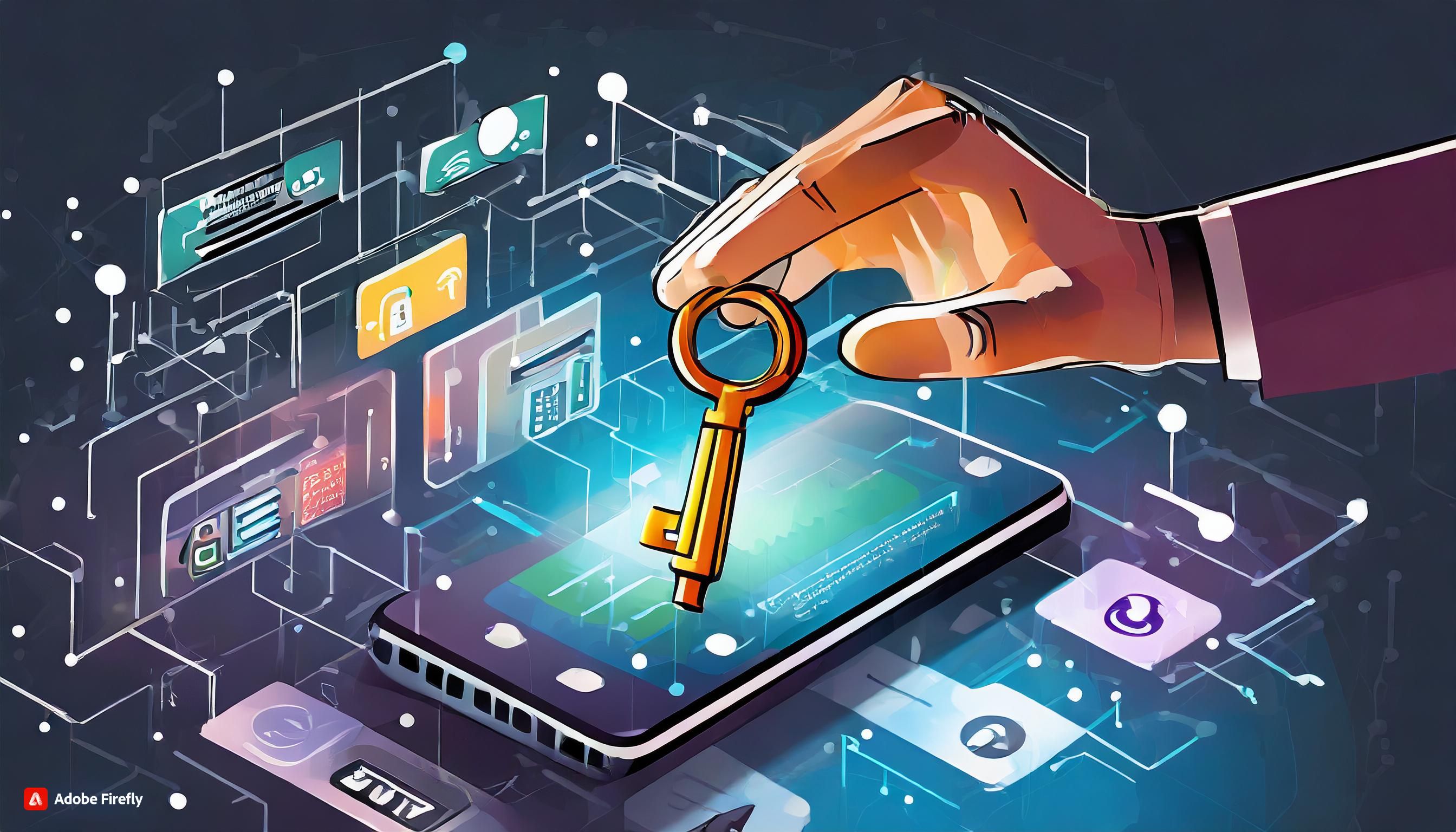
Introduction:
Recently I came across a problem submitting a form on a static Astro site to a CRM. The site requested a key for submission, exposing this client-side is a big security risk. That’s where serverless functions come into play. In this blog post, I’ll walk you through how to create a Vercel serverless function to securely handle form submissions while keeping API keys hidden.
Steps to follow
Set Up Your Vercel Project If you haven’t already, sign up for a Vercel account and create a new project. You can either link your project to a Git repository or use Vercel’s CLI for local development.
Create a Serverless Function In your project directory, create a new directory named api to store your serverless functions. Inside the api directory, create a JavaScript file for your function. For example, let’s create submitForm.js.
export const config = {
runtime: "edge", // this is optional.
};
export async function POST(request) {
const url = new URL(${process.env.API_ENDPOINT});
let headers = {
Authorization: `Bearer ${process.env.API_KEY}`,
"Content-Type": "application/json",
Accept: "application/json",
};
try {
const requestBody = await request.json();
const email = requestBody.email;
const response = await fetch(url.toString(), {
method: "POST",
headers: headers,
body: JSON.stringify({
email: email,
}),
});
return new Response("Success!", { status: response.status });
} catch (error) {
console.error("Error:", error);
return new Response("Error!", { status: 500 });
}
}
Set Environment Variables To securely store your API key, you’ll need to define them as environment variables in your Vercel project. Navigate to your Vercel project dashboard and go to Settings > Environment Variables. Add the new environment variables named API_KEY and API_ENDPOINT then paste your keys as the value. Ensure that you keep your API key confidential and never expose it in your code or repositories.
Step 4: Deploy Your Project Once you’ve set up your serverless function and defined your environment variable, deploy your project to Vercel. Vercel will automatically deploy your serverless function and make it accessible via a unique endpoint.
Integrate the Function with Your Form Update your form’s submission logic to make a POST request to the endpoint of your serverless function. For example:
<form action="/api/submitForm" method="post">
<!-- Form fields -->
<button type="submit">Submit</button>
</form>
With these steps completed, your form submissions will be processed securely through your Vercel serverless function, with the API key hidden from client-side code.
We will just use a standard html form for the submission you can style this any way you like
Conclusion
Securing sensitive information like API keys is a critical aspect of web development. By leveraging Vercel’s serverless functions, developers can hide API keys from client-side code, reducing the risk of unauthorized access and potential security breaches. Implementing serverless functions not only enhances security but also improves scalability and maintainability.